Java Inheritance – In this tutorial, we learn how to create inheritance in Java and types of inheritance with examples.
What is Inheritance
It it concept of OOPs in which we can create a new class based upon existing class. The new class can inherit member of existing class in it. The new class is called sub, derived or child class. The existing class is called base, super or parent class. For example we have two classes Employee and Part time Employee, part time employee is also an employee. The relation between Employee and part Employee is inheritance.
Why Inheritance
The purpose of inheritance in Java is re-usability. It means methods and field of parent class can be reuse in child class.
Java Inheritance Syntax
In Java, Parent(super) class is derived by the child(sub) class using extends keyword. The syntax to create inheritance in java is below here.
class ParentClass
{
//members of the parent class.
}
class ChildClass extends ParentClass
{
//members of child class.
}
extends – It is a keyword in java, to create relationship(inheritance) between two classes.
Example of Multiple Classes (Without Inheritance)
class ParentClass
{
public void parentMethod()
{
System.out.println("Parent Class");
}
}
class ChildClass
{
public void childMethod()
{
System.out.println("Child Class");
}
public static void main(String args[])
{
ParentClass pc=new ParentClass();
ChildClass cc=new ChildClass();
pc.parentMethod();
cc.childMethod();
}
}
In the above example we have created two classes, but the main method should be one in any class. We have to create separate object of the classes and methods of the class is called with the reference of object of own class.
Example – How to create Inheritance
We will use above example to create inheritance in Java.
class ParentClass
{
public void parentMethod()
{
System.out.println("Parent Class");
}
}
class ChildClass extends ParentClass
{
public void childMethod()
{
System.out.println("Child Class");
}
public static void main(String args[])
{
ChildClass cc=new ChildClass();
cc.parentMethod();
cc.childMethod();
}
}
Some Important Points from the above example.
- we are using extends keyword for inheritance.
- You need not create object of parent class.
- You can access member of the parent class with the reference of the child class.
- This is the example of single inheritance.
Types of Inheritance in Java
OOPs has many types of inheritance, but java support few of them.
- Single Inheritance
- Multilevel Inheritance
- Hierarchical Inheritance
Single Inheritance
In a single Inheritance, one class can extends another class.
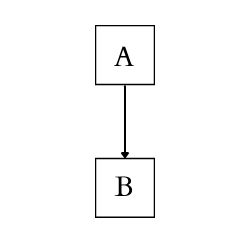
class A
{
public void methodA()
{
System.out.println("Parent class");
}
}
class B extends A
{
public void methodB()
{
System.out.println("Child class");
}
public static void main(String args[])
{
B b=new B();
b.methodA();
b.methodB();
}
}
Multilevel Inheritance
In multilevel Inheritance one child class can inherit from another child class.
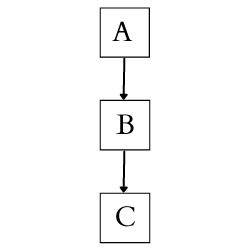
class A
{
public void methodA()
{
System.out.println("Parent class");
}
}
class B extends A
{
public void methodB()
{
System.out.println("Child class");
}
}
class C extends B
{
public void methodC()
{
System.out.println("Another Child class");
}
public static void main(String args[])
{
C c=new C();
c.methodA();
c.methodB();
c.methodC();
}
}
Hierarchical Inheritance
In Hierarchical Inheritance, one class can be inherited by many child class.
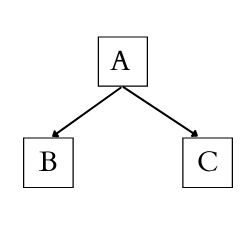
Java does not support multiple inheritance, so java is not a pure object oriented programming language.
Method Overriding in Java
When two classes have same method name and both class have relationship (Inheritance). Or you can say when a method of super class is redefine in sub class , it is known as method overriding.
Rules for Method Overriding
- There must be Inheritance between two classes.
- Method name must be same in both class.
- Method of child class must have same parameters as Parent class.
class A
{
//method of parent class
public void msg()
{
System.out.println("Parent class");
}
}
class B extends A
{
//method overriding
//same name and parameter as parent class
public void msg()
{
System.out.println("Child class");
}
public static void main(String args[])
{
B b=new B();
b.msg();
}
}
Child class
As we know we can call method of parent class with the help of the object of child class. In the above example only method of child class will call.
super keyword in Java
It is a keyword in java and referred as a reference object of parent class. It is also used to call member of parent class in child class.
class A
{
//method of parent class
public void msg()
{
System.out.println("Parent class");
}
}
class B extends A
{
//method overriding
public void msg()
{
super.msg(); //calling method of parent class.
System.out.println("Child class");
}
public static void main(String args[])
{
B b=new B();
b.msg();
}
}
Parent class
Child class